yield return and IEnumerable
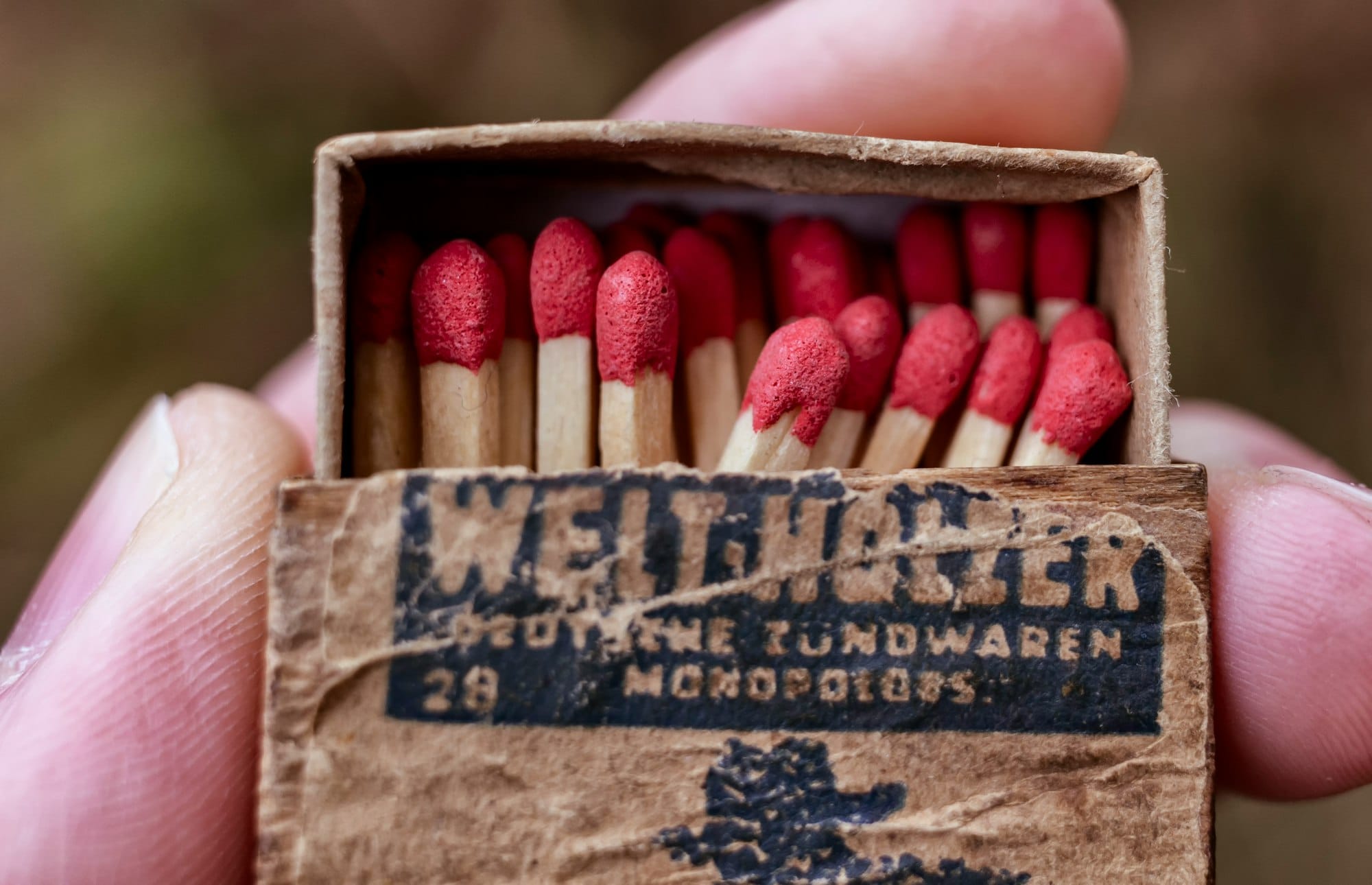
Using yield return
in C# can be quite powerful in certain scenarios but comes with trade-offs. Here’s a breakdown of when it’s useful and when it might not be ideal:
When to Use yield return
- When You Want Lazy Evaluation (Deferred Execution):
- Scenario: You have a large collection or expensive-to-compute data, but you don't need to retrieve all items at once.
- Example: Iterating through a potentially infinite sequence, or when you only need a few items from the beginning of a large collection.
- Benefit:
yield return
produces elements one at a time as they are requested, so memory consumption is minimized, and expensive computations are deferred until necessary. - Use Case
IEnumerable<int> GenerateNumbers()
{
for (int i = 0; i < int.MaxValue; i++)
{
yield return i;
}
}
Here, only the numbers that are iterated over are actually computed, rather than all numbers upfront.
- When the Sequence is Computed Dynamically:
- Scenario: The sequence depends on a computation or data source that changes over time.
- Example: Data from a database or an API that might change between iterations.
- Benefit:
yield return
allows you to compute elements lazily, so the most up-to-date data can be fetched during iteration. - Use Case
IEnumerable<string> FetchDataFromApi()
{
// Fetch data chunk by chunk lazily as requested.
foreach (var item in GetApiData())
{
yield return item;
}
}
- Reducing Memory Footprint for Large Datasets:
- Scenario: The data set is too large to hold in memory all at once.
- Example: Processing log files line by line, where the entire file would be too large to fit into memory.
- Benefit: Instead of loading the entire dataset into memory,
yield return
lets you process one item at a time, keeping memory usage low. - Use Case
IEnumerable<string> ReadLargeFile(string filePath)
{
using (var reader = new StreamReader(filePath))
{
while (!reader.EndOfStream)
{
yield return reader.ReadLine();
}
}
}
- When Writing Custom Iterators:
- Scenario: You need custom logic for iteration, and
yield return
makes it easy to control the iteration flow. - Example: Filtering, transforming, or producing elements dynamically during iteration without manually creating an
IEnumerator
. - Benefit: Simplifies writing custom iterators and hides the complexity of managing state between iterations.
- Use Case
- Scenario: You need custom logic for iteration, and
IEnumerable<int> GetEvenNumbers(int max)
{
for (int i = 0; i <= max; i++)
{
if (i % 2 == 0)
yield return i;
}
}
When Not to Use yield return
- When Immediate Access to All Elements is Required:
- Scenario: You need all elements immediately, either to perform an operation on them or to iterate multiple times.
- Example: You want to run a
ToList()
orToArray()
on the result of theIEnumerable
returned byyield return
, which would negate the benefit of deferred execution. - Downside: If you need to materialize the entire sequence (e.g.,
ToList()
), usingyield return
is inefficient because the whole sequence will be computed anyway, and the deferred execution overhead adds complexity.
- Performance-Critical Sections:
- Scenario: In tight loops or performance-critical sections where each iteration needs to be as fast as possible.
- Example: Real-time applications or hot paths in the code where any additional overhead from deferred execution would degrade performance.
- Downside:
yield return
introduces some overhead due to state management of the enumerator, which may not be suitable for scenarios that demand high performance or low-latency execution.
- When the Sequence is Small and Known Upfront:
- Scenario: You have a small, fixed collection that can be easily materialized in memory.
- Example: Small arrays or lists that don’t justify the complexity and overhead of
yield return
. - Downside: The simplicity and predictability of using a
List<T>
or array is preferable, as it avoids the overhead of state machine creation that comes withyield return
.
- Complex State Management:
- Scenario: When the logic inside the iterator becomes complex, with multiple nested loops or conditions that make the control flow harder to follow.
- Example: Custom iterators with complex branching or recursion may become difficult to reason about with
yield return
. - Downside: Writing custom stateful iteration logic can be tricky to debug, and the code might become harder to maintain.
- When You Need to Iterate Multiple Times:
- Scenario: You need to iterate the collection multiple times, but
yield return
recreates the sequence each time it’s iterated. - Example: With
List<T>
, you can iterate over the list as many times as needed without re-executing the logic. Withyield return
, each iteration re-executes the generator logic. - Downside: Repeatedly generating the sequence can be inefficient if multiple iterations are required.
- Scenario: You need to iterate the collection multiple times, but
Summary Table
Use Case | When to Use yield return | When to Avoid yield return |
---|---|---|
Lazy evaluation / deferred execution | Useful when you only need parts of a sequence at a time. | Avoid when you need all elements immediately. |
Handling large datasets | Use when processing large datasets that cannot fit into memory. | Avoid for small datasets or when everything must be materialized. |
Memory efficiency | Great for reducing memory consumption in resource-constrained apps. | Avoid when you need fast access to all elements at once. |
Custom iterators | Simplifies custom iteration logic. | Complex state management can make debugging difficult. |
Performance-sensitive applications | Avoid in tight loops or hot paths due to overhead. | Use List<T> for faster access and iteration. |