String Interning in C#
String interning is a technique used in C# to improve the efficiency of string comparison and manipulation. It allows strings that have the…
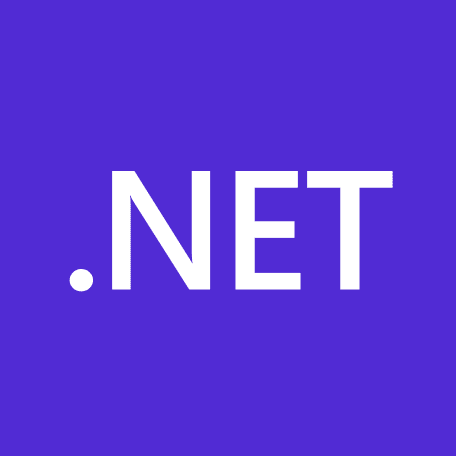
String interning is a technique used in C# to improve the efficiency of string comparison and manipulation. It allows strings that have the same value to be stored in the same location in memory, reducing the amount of memory used by the application and improving performance.
When a string is created in C#, it is usually allocated in memory on the heap. However, if the string is interned, it is stored in a special table called the intern pool. The intern pool is a table of unique strings that are shared throughout the application.
To intern a string in C#, you can use the String.Intern() method. This method returns a reference to the interned string if it already exists in the intern pool, or it adds the string to the intern pool and returns a reference to it.
Here’s an example of how to use String. Intern to intern a string:
string s1 = "hello";
string s2 = String.Intern("hello");
Console.WriteLine(object.ReferenceEquals(s1, s2)); // prints "True"
In this example, s1 and s2 both contain the same value, “hello”. However, s1 is allocated on the heap, while s2 is interned and stored in the intern pool. The object.ReferenceEquals method is used to compare the references of s1 and s2, and it returns true because they both refer to the same object in memory.
Interning strings can be useful in scenarios where you need to compare strings frequently, such as in a loop or in a search algorithm. By interning the strings, you can reduce the amount of memory used by the application and improve performance.
However, it’s important to note that string interning is not always the best approach. If you’re working with a large number of unique strings, or if the strings are only used once or twice, interning may not provide any benefit and may even degrade performance. In addition, interned strings are not garbage collected until the application exits, so if you’re interning a large number of strings, you may end up with a large memory footprint.
In conclusion, string interning is a technique used in C# to improve the efficiency of string comparison and manipulation. By storing strings with the same value in the intern pool, you can reduce the amount of memory used by the application and improve performance. However, it’s important to use string interning judiciously and only in scenarios where it provides a clear benefit.