Code as data and AI-Based Modernization
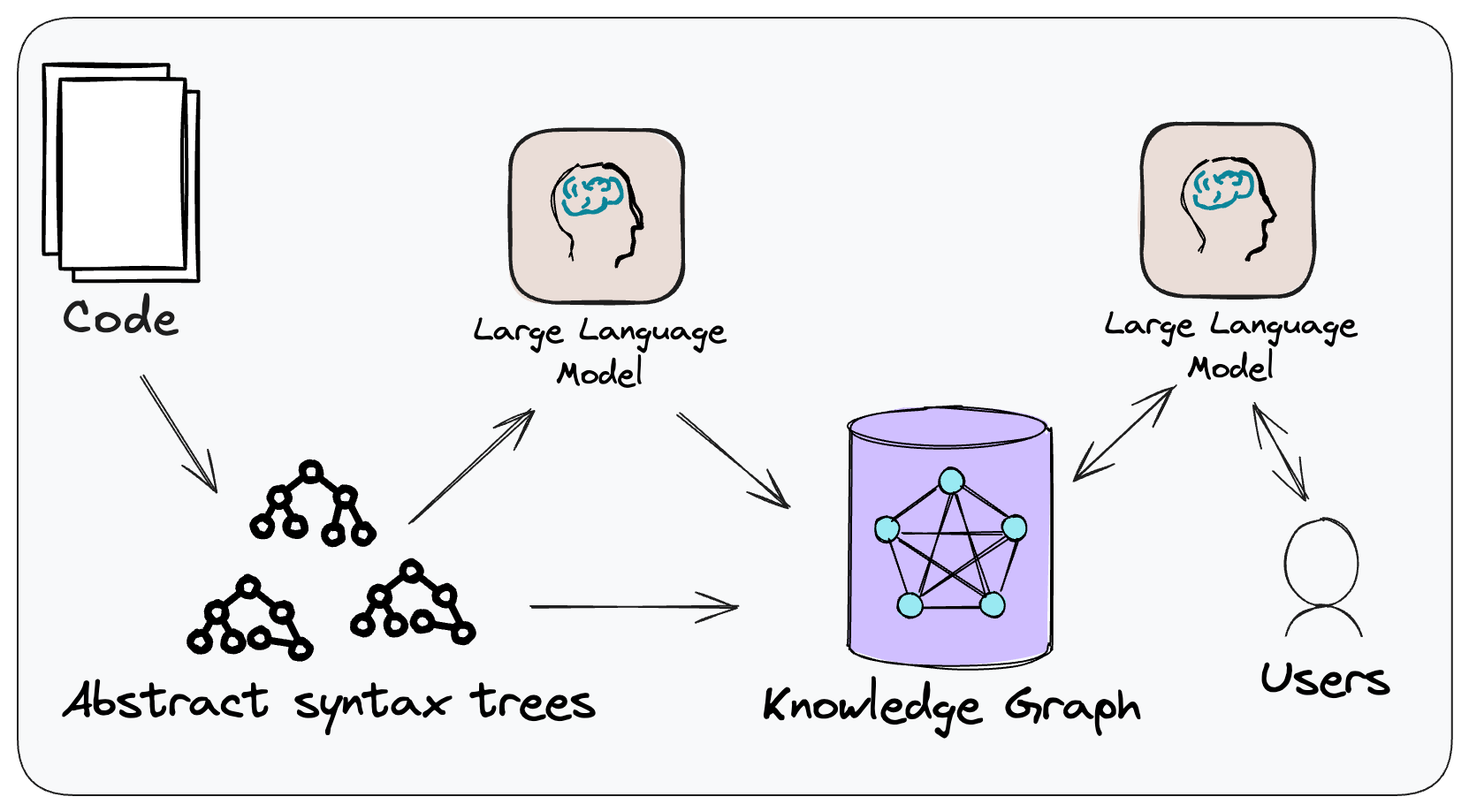
"Code as data" refers to treating source code as manipulable data, which enables systems to analyze, interpret, and transform it much like other forms of structured information. This concept is key in advanced tools for software modernization, where abstract syntax trees (ASTs) are used to represent code in a structured format, breaking it down into nodes that reflect the syntax and structure of the program. ASTs allow detailed analysis of the code, such as identifying dependencies between classes, methods, and properties.
By storing these relationships in a graph database like Neo4j, developers can visualize and traverse the codebase in a manner that is more meaningful and efficient for large-scale analysis. Graphs model complex interactions between different elements of the code, making it easier to extract high-level abstractions and insights from the codebase, such as dependencies and interactions between different components.
GraphRAG, an approach where retrieval-augmented generation (RAG) integrates graph traversal techniques, takes this further by leveraging knowledge graphs in AI-powered systems. In a GenAI environment, such as those used in modernization efforts, these graphs enhance the performance of large language models (LLMs) by providing contextual information about the codebase. This enables LLMs to generate explanations, translate code across languages, and provide insights into both low-level implementation details and high-level system architecture.
The combination of ASTs, Neo4j, and GenAI helps organizations modernize their legacy systems, efficiently migrate code, and improve understanding of complex codebases through graph-based insights and AI-driven analysis.
Here’s an example that integrates the concepts of Code as Data, AST, Neo4j, and GraphRAG into a C# workflow. This code reads .cs
files, extracts some basic structure from them (like classes, methods, and attributes), and stores this information into a Neo4j graph database.
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Microsoft.CodeAnalysis;
using Microsoft.CodeAnalysis.CSharp;
using Neo4j.Driver;
class Program
{
static async Task Main(string[] args)
{
string csFilePath = @"C:\Path\To\Your\CsFiles";
var files = Directory.GetFiles(csFilePath, "*.cs", SearchOption.AllDirectories);
using var driver = GraphDatabase.Driver("bolt://localhost:7687", AuthTokens.Basic("username", "password"));
using var session = driver.AsyncSession();
foreach (var file in files)
{
string code = File.ReadAllText(file);
SyntaxTree tree = CSharpSyntaxTree.ParseText(code);
var root = (CompilationUnitSyntax)tree.GetRoot();
var classDeclarations = root.DescendantNodes().OfType<ClassDeclarationSyntax>();
foreach (var classDeclaration in classDeclarations)
{
string className = classDeclaration.Identifier.Text;
var attributes = classDeclaration.AttributeLists.SelectMany(a => a.Attributes).Select(attr => attr.ToString());
await session.RunAsync($"CREATE (c:Class {{name: '{className}'}})");
// Store attributes of the class in Neo4j
foreach (var attribute in attributes)
{
await session.RunAsync($"MATCH (c:Class {{name: '{className}'}}) CREATE (c)-[:HAS_ATTRIBUTE]->(:Attribute {{name: '{attribute}'}})");
}
// Extract and store methods
var methods = classDeclaration.DescendantNodes().OfType<MethodDeclarationSyntax>();
foreach (var method in methods)
{
string methodName = method.Identifier.Text;
await session.RunAsync($"MATCH (c:Class {{name: '{className}'}}) CREATE (c)-[:HAS_METHOD]->(:Method {{name: '{methodName}'}})");
// Example: Store method parameters or dependencies (like class Person)
var parameters = method.ParameterList.Parameters.Select(p => p.Identifier.Text);
foreach (var parameter in parameters)
{
await session.RunAsync($"MATCH (m:Method {{name: '{methodName}'}}) CREATE (m)-[:HAS_PARAMETER]->(:Parameter {{name: '{parameter}'}})");
}
}
}
}
}
}